Android App Development: Essential Guide to Creating Successful Mobile Applications
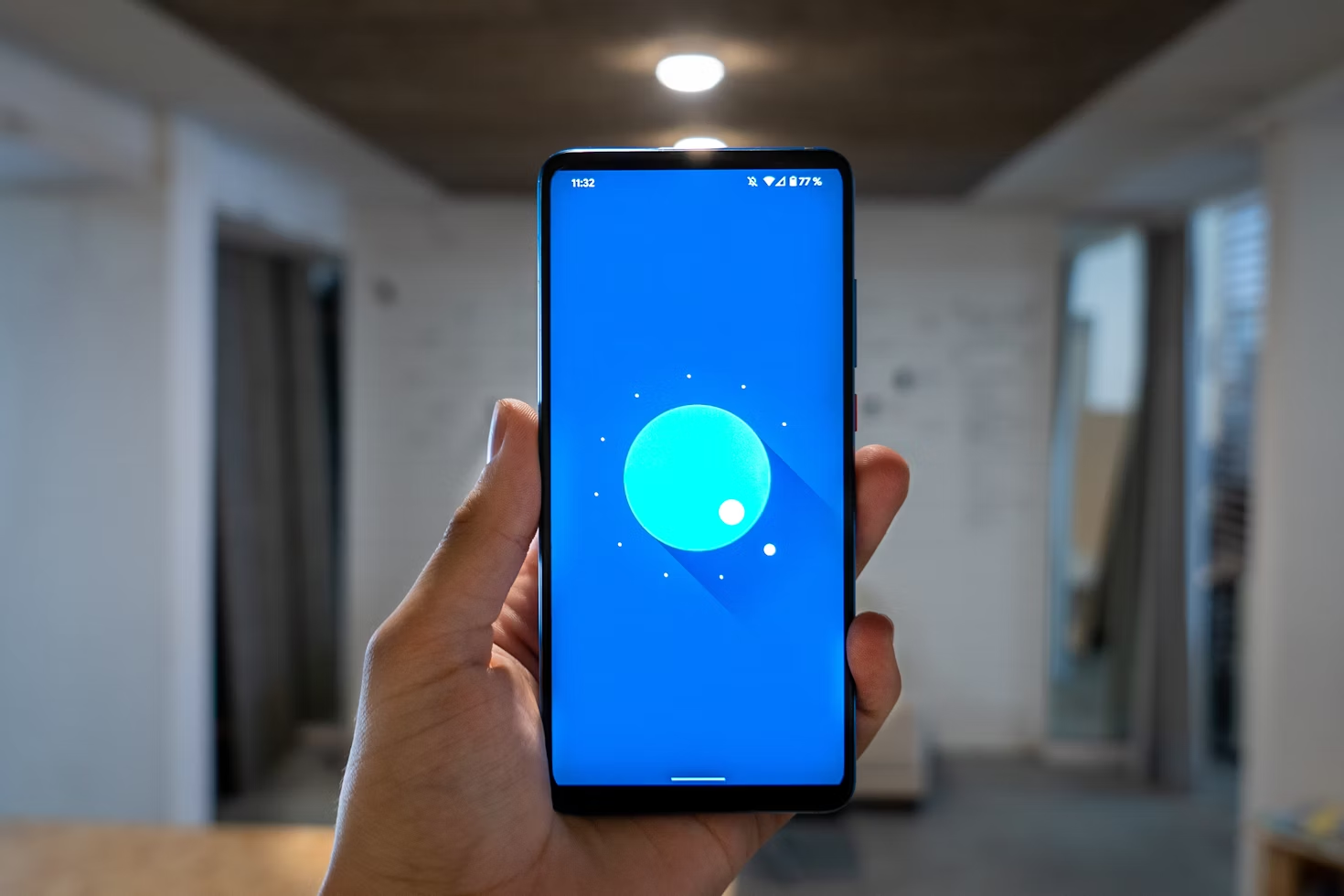
Developing an Android app can seem challenging, but it is a rewarding process that opens many opportunities. You can create apps that meet specific needs and engage millions of users around the world. With the right tools and knowledge, you can turn your ideas into a successful application.
This blog post will guide you through the essential steps of Android app development. You will learn about the best programming languages, necessary frameworks, and key features to include in your app. Whether you are a beginner or have some experience, understanding these elements is crucial for making your app stand out.
By the end of this article, you will have a clearer vision of how to start your Android app development journey. The knowledge you gain here will empower you to bring your app idea to life and potentially reach a wide audience.
Fundamentals of Android Architecture
Android architecture consists of key components that work together to create apps. Understanding these elements can help you design and build effective Android applications.
Understanding the Android Stack
The Android stack includes various layers that support app development. At the base is the Linux kernel, which provides core system services like security and memory management. Above the kernel, the hardware abstraction layer (HAL) allows the software to communicate with hardware components.
The Android Runtime (ART) follows, providing essential libraries and frameworks to run Android apps. On top of ART is the Application Framework, which allows developers to interact with system services and manage app components.
The Applications Layer is where your apps reside. This structure ensures that the system runs smoothly, enabling efficient app performance.
The Importance of the Android Manifest
The Android Manifest is a crucial XML file for every Android app. It declares essential information about the app, including its components, permissions, and minimum API level requirement.
Key elements in the manifest include:
- Activities:Defines the user interface screens of your app.
- Services:Specifies background processes.
- Content Providers:Outlines data sharing methods.
- Broadcast Receivers:Manages system-wide messages.
By clearly outlining these components, the manifest helps the Android operating system understand how to launch and manage your app, ensuring smooth operation and compliance with system policies.
Activity Lifecycle and Task Management
Understanding the Activity Lifecycle is vital for Android app development. An activity represents a single screen with a user interface, and it goes through several states: created, started, resumed, paused, stopped, and destroyed.
For effective task management, you need to know how to handle transitions between these states. Here are some key methods:
- onCreate(): Initialize your activity.
- onStart(): Make your app visible.
- onResume(): App is ready for user interaction.
- onPause(): App loses focus but may still be running.
- onStop(): App is no longer visible.
Managing the lifecycle helps maintain app performance, save resources, and provide a smooth user experience.
Setting Up the Development Environment
To begin developing Android apps, you need to set up your development environment. This includes choosing an Integrated Development Environment (IDE), configuring the Android SDK, and setting up testing options for your apps.
Choosing an IDE: Android Studio and Alternatives
Android Studio is the official IDE for Android development. It offers features like code editing, debugging, and performance tools. You can download it from the Android developer website.
If you prefer alternatives, consider IntelliJ IDEA or Eclipse. Both can be used for Android development, but they may not have all the features of Android Studio. Make sure to choose an IDE that suits your workflow and personal preferences to increase your productivity.
Configuring the Android SDK
After selecting your IDE, you need to install and configure the Android Software Development Kit (SDK). The SDK contains essential tools and libraries for building Android apps.
You can find the SDK in the Android Studio installation. To install it, open Android Studio, go to “SDK Manager,” and select the latest SDK version.
Make sure to install the necessary packages like SDK Build Tools, SDK Platform Tools, and the Android Emulator. These tools allow you to compile and run your apps efficiently.
Utilizing Virtual Devices and Real Device Testing
Testing your apps is crucial for ensuring quality. You can use the Android Emulator for virtual testing. It mimics a real Android device on your computer to simulate different screen sizes and operating systems.
To set up an emulator, open Android Studio, go to “AVD Manager,” and create a new virtual device.
In addition, testing on a real device provides a better experience. For this, enable Developer Options on your Android device. Then connect it to your computer with a USB cable and make sure USB debugging is turned on. This allows you to install and run apps directly on your device for real-world testing.
Design Principles for Android Apps
Creating an effective Android app requires understanding key design principles. This section explains important guidelines like Material Design, responsive layouts, and navigation patterns.
Material Design Guidelines
Material Design is a visual language developed by Google. It emphasizes clean, bold visuals to enhance user experience. You should use grid-based layouts to ensure consistency across screens.
Key Features:
- Color Palettes:Choose colors that work well together for a pleasing look.
- Typography:Use clear, readable fonts. Limit font types to improve clarity.
- Iconography:Use simple icons that convey meaning quickly and easily.
Make sure to follow these guidelines to provide a cohesive and user-friendly experience.
Responsive Layouts and Density Independence
Responsive layouts adjust to different screen sizes and orientations. This ensures your app looks good on various devices. Use flexible grids and scalable images for better adaptability.
Tips for Implementation:
- Density Independence:Use dip units to ensure elements scale correctly on all devices.
- Flexible Layouts:Implement ConstraintLayout or RelativeLayout to respond to screen changes.
- Testing:Always test on different device sizes to ensure your app performs well.
These practices help maintain usability and appearance across devices.
Navigation Patterns
Effective navigation is crucial for user satisfaction. Choose a pattern that suits your app’s purpose. This makes it easy for users to find what they need.
Common Navigation Methods:
- Bottom Navigation:Good for apps with three to five top-level destinations.
- Navigation Drawer:Useful for more complex apps with multiple sections.
- Tabs:Ideal for switching between related views quickly.
Ensure that navigation is intuitive. Users should be able to move through your app without confusion.
Android Programming Basics
Understanding Android programming is crucial for building effective applications. You will primarily use Kotlin or Java, focus on core components like activities and fragments, and manage app resources efficiently.
Kotlin and Java Language Features
Kotlin is now the preferred language for Android development because of its modern features. It is concise and helps reduce boilerplate code, making your code easier to read. Key features of Kotlin include:
- Null Safety:Helps prevent null pointer exceptions.
- Extension Functions:Allows you to add new functionality to existing classes.
- Coroutines:Simplifies asynchronous programming.
Java remains important as many legacy apps are built with it. It offers a robust library and has strong community support. Key elements of Java include:
- Strongly typed language, ensuring type safety.
- Object-oriented structure for organizing code.
- Extensive documentation and resources available online.
Core Components: Activities and Fragments
Activities are crucial in Android apps; they represent a single screen. Each activity can manage its user interface and handle user interactions. Key points about activities:
- Lifecycle Management:Activities have a lifecycle that controls how they are created and destroyed.
- Intents:Use intents to start activities or pass data between them.
Fragments are components that can be combined within activities. Fragments break down UI components into reusable pieces. Important aspects to consider:
- Modularity:You can use fragments for different layouts on various screen sizes.
- Fragment Lifecycle:Fragments have their own lifecycle but also depend on the parent activity.
Managing App Resources and Assets
Efficiently managing resources and assets is essential for a smooth user experience. Resources include images, layouts, strings, and more. Key resource types are:
- Drawable Resources:Store images and backgrounds.
- Layout Resources:Define the UI structure with XML files.
Organizing resources in different folders helps in supporting various device configurations. Use resource qualifiers like -land for landscape layouts or -v21 for features available in specific Android versions.
Assets are files you can include in your app, like fonts or sound files. You can access them through the assets folder. This management ensures your app is flexible and works well across devices.
Data Management
Efficient data management is crucial in Android app development. You will use various methods to store and access data, ensuring your app runs smoothly and meets user needs.
SQLite Databases and Room Persistence Library
SQLite is a lightweight database built into Android. It allows you to store structured data in tables. You can perform operations like insert, update, and delete using SQL queries.
The Room Persistence Library simplifies database management. It provides an abstraction layer over SQLite. This makes it easier to work with databases and reduces boilerplate code. Room handles relationships between data entities well and supports LiveData and RxJava for data observation.
To use Room, define data entities as Java or Kotlin classes. Create a database class that extends RoomDatabase. Then, use DAOs (Data Access Objects) to define methods for accessing the data. This setup makes managing your app’s data easier and more efficient.
Shared Preferences and Data Caching
Shared Preferences is a simple way to store small amounts of key-value data. You can use it for user settings or app preferences. This approach is ideal for data that doesn’t need complex structures.
To use Shared Preferences, call getSharedPreferences() and access the data using methods such as getString() or putInt(). Data stored here persists even after the app is closed.
Data caching improves performance by storing temporary data. You can use it to keep data that users frequently access. Caching reduces loading times and saves bandwidth. Storing images or other large files in cache can be beneficial for quick access.
Content Providers and Resolvers
Content Providers enable you to share data between applications in a structured way. They act as an interface for accessing data, whether it’s from your app or another app.
Implementing a Content Provider involves extending the ContentProvider class. You must define methods for CRUD operations (Create, Read, Update, Delete). This allows other apps to access your data securely.
To access data from a Content Provider, use a Content Resolver. You can query, insert, update, or delete data through this interface. Using Content Providers enhances data sharing and collaboration between different applications.
User Interface Development
Creating a user-friendly interface is essential in Android app development. This section focuses on building layouts, designing custom views, and adding animations to improve user experience.
Building Layouts with XML
In Android development, you use XML to define your app’s layout. XML allows you to create a structured way to organize UI elements like buttons, text fields, and images. To start, you typically use a layout manager, such as LinearLayout or ConstraintLayout.
Key Attributes:
- Width and Height:Set the size of the elements. Use match_parent or wrap_content.
- Gravity:Align elements within their container.
- Padding and Margin:Adjust space around and within elements.
Example layout for a simple screen:
android:layout_width=”match_parent” android:layout_height=”match_parent” android:orientation=”vertical”>
Custom Views and Canvas Drawing
For unique designs, you may need to create custom views. This involves extending existing view classes or creating new ones. By overriding the onDraw method, you can use the Canvas class to draw shapes and images.
Key Steps:
- Extend a View Class:Use View, SurfaceView, or others based on your needs.
- Override onDraw():Use Canvas methods like drawRect() or drawBitmap() to render graphics.
- Handle Touch Events:Add interactivity by overriding touch handling methods.
Here’s a simple example of a custom view:
public class MyCustomView extends View {
protected void onDraw(Canvas canvas) {
Paint paint = new Paint();
paint.setColor(Color.BLUE);
canvas.drawRect(50, 50, 200, 200, paint);
}
}
Animating UI Components
Animations enhance user experience by making interactions feel smooth and appealing. In Android, you can use the Animation class or Transition API for animating UI components.
Common Animation Types:
- View Animation:Fades, rotates, or moves views.
- Drawable Animation:Changes images in an AnimationDrawable.
- Property Animation:Allows you to animate any property, like size or color.
Example of a simple fade-in animation:
android:fromAlpha=”0.0″ android:toAlpha=”1.0″ android:duration=”500″/> Use animations thoughtfully for a better user experience without overwhelming the user. App Functionality Enhancement Enhancing app functionality is crucial for providing a better user experience. This can be achieved through various methods, such as implementing background services, using broadcast receivers, and scheduling tasks. Implementing Background Services Background services allow your app to perform operations even when the user is not actively using it. This feature is essential for tasks like downloading files or syncing data. To create a background service, extend the Service class. You will need to override methods like onStartCommand(). Use the startService() method to begin your service. It’s important to manage resources properly. For instance, if your service runs too long, it can drain battery life. You can also use JobIntentService for better management of background tasks. Using Broadcast Receivers Broadcast receivers help your app listen for specific events from the system or other apps. This is useful for responding to actions like connectivity changes or incoming messages. To create a broadcast receiver, extend the BroadcastReceiver class. Override the onReceive() method to define what your app should do when it receives a broadcast. Register your receiver in the AndroidManifest.xml or in your code. It’s also important to unregister receivers to prevent memory leaks. You can use local broadcasts for tighter control over communication within your app. Scheduling Tasks with WorkManager WorkManager is a powerful tool for scheduling tasks that need to run even if your app is not currently active. It is suitable for background tasks that are guaranteed to execute. To use WorkManager, create a Worker class and define the doWork() method. You can then schedule your worker with OneTimeWorkRequest or PeriodicWorkRequest. WorkManager intelligently decides how to run your tasks based on constraints like network availability or battery level. This way, you optimize app performance while remaining efficient with resources. Connectivity and Networking Connectivity is crucial for Android app development. It allows users to communicate with servers and access the internet. You will learn about popular libraries for networking, methods for real-time communication, and handling network states. Networking in Android with Retrofit and OkHttp Retrofit and OkHttp are popular libraries for networking in Android. Retrofit simplifies API calls and data conversion with ease. It uses annotations to manage requests and responses. OkHttp is the underlying client for making HTTP requests. It manages connection pooling, caching, and asynchronous requests efficiently. Together, they streamline the process of network communication. Using these libraries, you can create responsive and efficient apps. WebSockets and Real-time Communication WebSockets enable real-time communication between clients and servers. They allow for full-duplex communication, meaning both parties can send and receive messages simultaneously. To implement WebSockets in your app, you can use libraries like Retrofit or libraries specifically for WebSockets. Managing connections and data efficiently is key. This ensures your app remains responsive and quick. Handling Network State and Permissions Managing network state is important for user experience. You need to check if the device is connected to the internet before making requests. Permissions are also essential. Your app must request the necessary permissions to access the internet. Handling these factors helps maintain a smooth experience in your Android app. Keeping users informed about their network status can enhance usability. Advanced Android Features This section covers important advanced features in Android development that enhance user experience. You will learn about integrating Google Play Services, utilizing location and map services, and incorporating sensors and device hardware. Integrating Google Play Services Google Play Services provides essential tools for app developers. This includes features such as Google Maps, Gmail, and Google Drive. To integrate these features, you need to add dependencies to your project. This is done in the build.gradle file. After that, you can access APIs by initializing the Google API Client. Location and Map Services Location and map services are crucial for apps that need to track user location or display maps. You can use the Google Maps API for detailed mapping functions. To implement these features, start by setting up the Maps SDK for Android. Ensure you have the necessary permissions to access the user’s location. Sensor Integration and Device Hardware Android devices come with various sensors like accelerometers, gyroscopes, and GPS. By tapping into these sensors, you can create interactive experiences. To utilize these sensors, you will work with the SensorManager class. Ensure that you handle sensor data efficiently to maintain app performance. Quality Assurance Quality assurance is crucial in Android app development. It ensures your app functions correctly, provides a good user experience, and performs efficiently. Below are key areas to focus on. Writing and Running Unit Tests Unit testing is essential to verify that individual components of your app work as intended. You can use frameworks like JUnit or Robolectric for this purpose. Create test cases for different scenarios to check edge cases and error handling. It’s important to write tests early in the development process. Running these tests frequently helps catch bugs before they escalate. Aim for at least 80% code coverage to ensure most functionalities are tested. Automating your unit tests saves time and reduces manual errors. Run them every time you make changes to maintain high code quality. UI Testing with Espresso UI testing is necessary to validate the app’s user interface and interactions. Espresso is a popular framework that helps you test UI components effectively. With Espresso, you can simulate user actions, like clicks and text entries. This framework waits for your app to be idle before running tests, which helps ensure reliability. Write tests for different devices and screen sizes to ensure a consistent experience. Use the Espresso Test Recorder to quickly generate test code based on user interactions. Regularly testing the UI helps identify issues with layout, navigation, and responsiveness before release. Performance Monitoring and Optimization Monitoring your app’s performance is key to providing a smooth experience. Use tools like Android Profiler to analyze CPU, memory, and network usage. Identify slow functions and optimize code to improve speed. Consider using asynchronous tasks to keep the app responsive during heavy operations. Regularly test how your app performs under different conditions, such as low battery or poor network connectivity. Optimize images and reduce app size to enhance loading times. Ensure your app runs efficiently on various devices to reach a broader audience. App Distribution and Monetization Distributing your app and finding ways to make money from it are two important steps. You can publish your app on the Google Play Store, add in-app purchases, or set up advertisements to earn revenue. Publishing to Google Play Store To publish your app, you first need a Google Play Developer account. This account costs a one-time fee of $25. You then prepare your app by following Google’s guidelines. You must provide details like the app’s title, description, and screenshots. It’s important to categorize your app correctly so users can find it easily. After submitting, your app goes through a review process. It can take a few hours to several days for approval. Once approved, users can download your app. You can also track downloads and user feedback through the Play Store Console. By responding to reviews and updating your app, you can improve user experience and retention. Implementing In-app Purchases In-app purchases allow users to buy extra features or content within your app. To add this, you’ll need to set up Google Play Billing. This service handles transactions safely for you. You can offer different types of purchases: consumables (like coins), non-consumables (like premium features), and subscriptions. Make sure to clearly explain what users get after making a purchase. Pricing should reflect the value you provide. Monitor user behavior to adjust prices or add new items. Clear communication about transactions helps build trust with your users. Setting Up App Advertisements Monetizing through ads is another way to earn money. You can use ad networks like AdMob to display ads in your app. Choose between banner ads, interstitial ads, or rewarded video ads to fit your app’s design. Ensure ads do not disrupt user experience. Balance ad placements to keep users engaged. You can also select specific audiences for targeted ads to increase click-through rates. Track your ad performance regularly. Understanding user interactions with ads helps in maximizing earnings. Adjust your ad strategy based on this data to improve revenue without harming user satisfaction. Post-Release Support and Maintenance Post-release support and maintenance are crucial for the success of your Android app. You need to ensure that your app remains functional and up to date. This involves regular updates, listening to user feedback, and maintaining a smooth deployment process. App Updates and Versioning You should plan regular app updates to fix bugs and improve features. Start with a versioning system to keep track of changes. Use semantic versioning, which follows a format like MAJOR.MINOR.PATCH. Make clear release notes for each update to inform users of what’s new. This builds trust and keeps users engaged. User Feedback and Crash Reporting Listening to user feedback is essential. Create channels for users to report issues and share suggestions. You can use tools like surveys or in-app feedback options. Crash reporting tools help you identify problems. Services like Firebase Crashlytics provide insights into crashes. This information is valuable for troubleshooting and enhancing user experiences. Responding to feedback quickly fosters a sense of community and encourages user retention. Continuous Integration and Deployment Implement continuous integration (CI) and continuous deployment (CD) to streamline updates. CI allows your development team to merge code changes regularly, leading to better quality. You can automate builds and tests with tools like Jenkins or CircleCI. This ensures that new updates do not break existing features. Continuous deployment helps you push updates smoothly and frequently. This keeps your app fresh and competitive. Ensure tests are in place to catch issues before deployment. Lastly, if you want to hire top tech talent for your app, I suggest you check Yeeply to help you find the best talents.